Section 2.6 Examples and questions
These are additional examples for reviewing the topic we have covered. When reading each example, try to find your own solution before clicking “Answer”. There are also questions for reviewing the concepts of this section.
Example 2.6.1. Tridiagonal matrix of arbitrary size.
diag(2*ones(1, n)) - diag(ones(1, n-1), 1) - diag(ones(1, n-1), -1)
or
2*diag(ones(1, n)) - diag(ones(1, n-1), 1) - diag(ones(1, n-1), -1)
or
2*eye(n) - diag(ones(1, n-1), 1) - diag(ones(1, n-1), -1)
It is important to recognize why n-1
appears above. The main diagonal has length n
but the diagonals next to it are shorter by 1 element, so their length is n-1
.
Example 2.6.2. Solving a tridiagonal system.
n = 28; A = 3*eye(n) - diag(ones(1, n-1), 1) - diag(ones(1, n-1), -1); b = zeros(n, 1); b(1) = 1; b(end) = 2; x = A\b; disp(x)
Explanation. The coefficient matrix has 3 on the main diagonal, and -1 above and below it. This matrix is created with the help of diag
as explained in Section 2.2. The right-hand size column vector begins with all-zeros: note that zeros(n, 1)
is a column of zeros, while zeros(1, n)
is a row of zeros. The first and last entries of b
are changed as described in the system.
Example 2.6.3. Table of squares and cubes of small integers.
The single line A = (1:9).^((1:3)')
does the job. The elementwise operation .^
can combine the arrays of sizes 1×9 and 3×1, as explained in Section 2.4. The result is a 3×9 matrix with the expected property: the base (1 through 9) is the column number, the exponent (1 through 3) is the row number.
Example 2.6.4. Plot of a function.
x = linspace(-1, 5, 500); y = (x - 1)./(x.^2 + 1); plot(x, y)
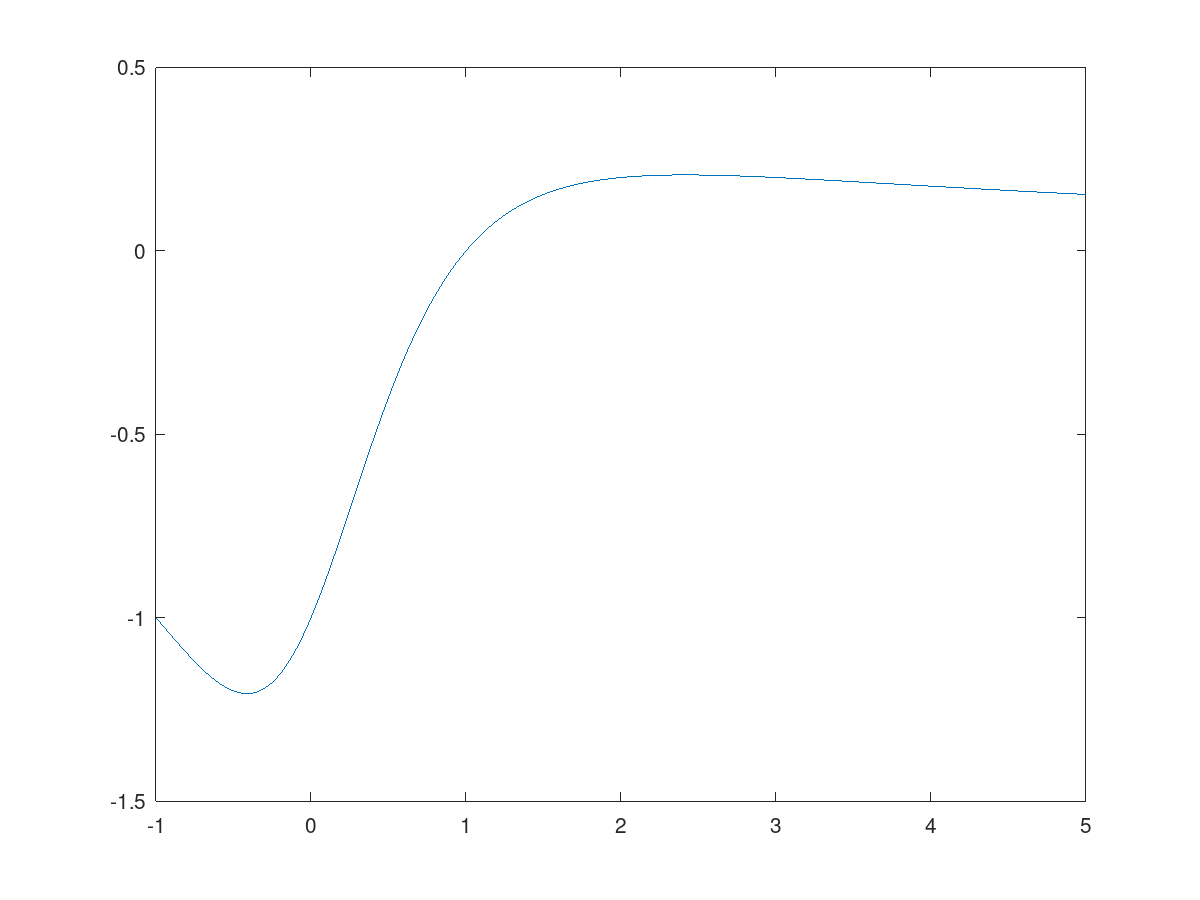
Note the use of semicolons to prevent Matlab from displaying hundreds of numbers that we do not need to see.
Question 2.6.6. x as a function of y.
How would you plot a function where \(x\) is given as a function of \(y\text{,}\) for example \(x = y + \cos y\) with \(-5\le y\le 5\text{?}\)
Question 2.6.7. A “wrong” way to multiply matrices.
Can you imagine a situation where you would want to compute A.*B
where both A
and B
are square matrices of the same size?
An idea: a matrix can be used to store a greyscale image, so that each entry is the brightness of the corresponding pixel. What would elementwise multiplication A.*B
mean in this context?